Sponsored Links
Ad by Google
In this post we are going to show you how to use Many-To-Many association with extra columns in hibernate using annotation. For Many-To-Many association example you can visit my previous post Many-To-Many association example using annotation or Many-To-Many association example using hbm.xml
Here is Many-To-Many association mapping table ER diagram.


Main Objects of this project are:
Step 1. Create database script.
Step 2. Create a Maven Project:
Step A: Go to File->New->Other..
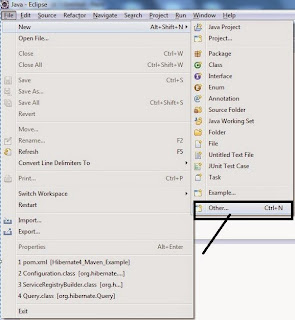
Step B: Select Maven Project from the select wizard.
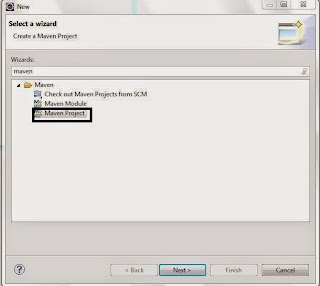
Step C: Select project name and location from New Maven Project wizard.
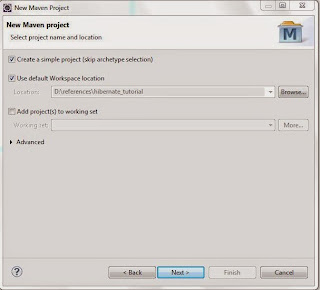
Step D: Configure project, provide GroupId, artifactId etc. See the details from the screenshot. This screen shot is from our previous post so here you need to change the artifactId as Many-To-Many-Association-Example.
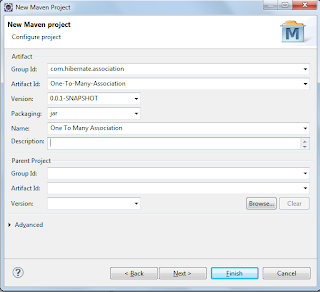
Step E: After completion of all the above steps, now your project will looks like this screenshot.

3. Add project dependencies into pom.xml file:
Double click on your project's pom.xml file it will looks like this with very limited information.
Now add Hibernate and MySql dependencies entry inside pom.xml file. Paste the below code inside the project tag of pom.xml file.
Here is a complete pom.xml file
4. Create a hibernate.cfg.xml file:
Create hibernate.cfg.xml file inside src/main/resources folder.
hibernate.cfg.xml
Step 5. Create an Employee annotated class:
Employee.java
Step 6. Create Project annotated class:
Project.java
Step 7: Create EmployeeProjectPK annotated class: EmployeeProjectPK is an Embeddable class.
EmployeeProjectPK.java
Step 8: Create EmployeeProject annotated class. For employee and project association table.
EmployeeProject.java
Step 9. Create a HibernateUtility class: HibernateUtility class to build SessionFactory via loading Configuration file.
Step 10. Create an EmployeeDAO class: To perform insert/update/get/delete records into the database.
EmployeeDAO.java
Step 11. Create an EmployeeService class: To call the methods of EmployeeDAO class to perform CRUD operations.
EmployeeService.java
OUT PUT:
Here is Many-To-Many association mapping table ER diagram.

Tools and Technologies we are using here:
- JDK 7
- Hibernate 4.3.7
- MySql 5.1.10
- Eclipse Juno 4.2
- Maven 3.2

Main Objects of this project are:
- pom.xml
- hibernate.cfg.xml
- annotated pojo
- database
Step 1. Create database script.
CREATE DATABASE /*!32312 IF NOT EXISTS*/`hibernate_tutorial` USE `hibernate_tutorial`; /*Table structure for table `employee` */ DROP TABLE IF EXISTS `employee`; CREATE TABLE `employee` ( `employee_id` int(11) NOT NULL AUTO_INCREMENT, `doj` date DEFAULT NULL, `first_name` varchar(255) DEFAULT NULL, `last_name` varchar(255) DEFAULT NULL, PRIMARY KEY (`employee_id`) ) ENGINE=InnoDB AUTO_INCREMENT=101 DEFAULT CHARSET=latin1; /*Table structure for table `project` */ DROP TABLE IF EXISTS `project`; CREATE TABLE `project` ( `project_id` int(11) NOT NULL AUTO_INCREMENT, `exp_end_date` date DEFAULT NULL, `project_name` varchar(255) DEFAULT NULL, `start_date` date DEFAULT NULL, PRIMARY KEY (`project_id`) ) ENGINE=InnoDB AUTO_INCREMENT=101 DEFAULT CHARSET=latin1; /*Table structure for table `employee_proj` */ DROP TABLE IF EXISTS `employee_proj`; CREATE TABLE `employee_proj` ( `employee_id` int(11) NOT NULL, `project_id` int(11) NOT NULL, `acitve` tinyint(1) DEFAULT NULL, `is_deleted` tinyint(1) DEFAULT NULL, PRIMARY KEY (`employee_id`,`project_id`), KEY `FK_qj7sartib5vld3q8mxqxrby17` (`project_id`), CONSTRAINT `FK_ochtcv9fmp6k1r653ri2oyq9` FOREIGN KEY (`employee_id`) REFERENCES `employee` (`employee_id`), CONSTRAINT `FK_qj7sartib5vld3q8mxqxrby17` FOREIGN KEY (`project_id`) REFERENCES `project` (`project_id`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Step 2. Create a Maven Project:
Step A: Go to File->New->Other..
Step B: Select Maven Project from the select wizard.
Step C: Select project name and location from New Maven Project wizard.
Step D: Configure project, provide GroupId, artifactId etc. See the details from the screenshot. This screen shot is from our previous post so here you need to change the artifactId as Many-To-Many-Association-Example.
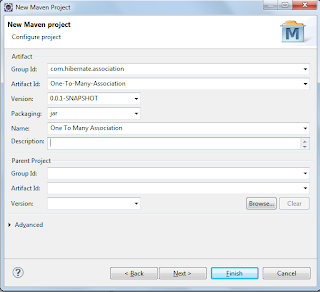
Step E: After completion of all the above steps, now your project will looks like this screenshot.

3. Add project dependencies into pom.xml file:
Double click on your project's pom.xml file it will looks like this with very limited information.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.hibernate.poc</groupId> <artifactId>Many-To-Many-Annotation-Example</artifactId> <version>0.0.1-SNAPSHOT</version> <name>Many-To-Many-Annotation-Example</name> </project>
Now add Hibernate and MySql dependencies entry inside pom.xml file. Paste the below code inside the project tag of pom.xml file.
<dependencies> <!-- Hibernate Dependency --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>4.3.7.Final</version> </dependency> <!-- MySql Connector dependency --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.10</version> </dependency> </dependencies>
Here is a complete pom.xml file
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.hibernate.poc</groupId> <artifactId>Many-To-Many-Annotation-Example</artifactId> <version>0.0.1-SNAPSHOT</version> <name>Many-To-Many-Annotation-Example</name> <dependencies> <!-- Hibernate Dependency --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>4.3.7.Final</version> </dependency> <!-- MySql Connector dependency --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.10</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.7</source> <target>1.7</target> </configuration> </plugin> </plugins> </build> </project>
4. Create a hibernate.cfg.xml file:
Create hibernate.cfg.xml file inside src/main/resources folder.
hibernate.cfg.xml
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/hibernate_tutorial</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <property name="dialect">org.hibernate.dialect.MySQL5InnoDBDialect</property> <property name="show_sql">true</property> <property name="format_sql">true</property> <property name="hbm2ddl.auto">update</property> <mapping class="com.hibernate.association.pojo.Employee"/> <mapping class="com.hibernate.association.pojo.Project"/> <mapping class="com.hibernate.association.pojo.EmployeeProject"/> </session-factory> </hibernate-configuration>
Step 5. Create an Employee annotated class:
Employee.java
package com.hibernate.association.pojo; import java.util.Date; import java.util.HashSet; import java.util.Set; import javax.persistence.CascadeType; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.FetchType; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.OneToMany; import javax.persistence.Table; import javax.persistence.Temporal; import javax.persistence.TemporalType; @Entity @Table(name = "employee") public class Employee { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) @Column(name = "employee_id") private int employeeId; @Column(name = "first_name") private String firstName; @Column(name = "last_name") private String lastName; @Column(name = "doj") @Temporal(TemporalType.DATE) private Date doj; @OneToMany(fetch = FetchType.LAZY, mappedBy = "id.employee", cascade = CascadeType.ALL) private Set<EmployeeProject> employeeProjects = new HashSet<EmployeeProject>(); public Set<EmployeeProject> getEmployeeProjects() { return employeeProjects; } public void setEmployeeProjects(Set<EmployeeProject> employeeProjects) { this.employeeProjects = employeeProjects; } public int getEmployeeId() { return employeeId; } public void setEmployeeId(int employeeId) { this.employeeId = employeeId; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public Date getDoj() { return doj; } public void setDoj(Date doj) { this.doj = doj; } @Override public String toString() { return "Employee [employeeId=" + employeeId + ", firstName=" + firstName + ", lastName=" + lastName + ", doj=" + doj + "]"; } }
Step 6. Create Project annotated class:
Project.java
package com.hibernate.association.pojo; import java.util.Date; import java.util.Set; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.FetchType; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.OneToMany; import javax.persistence.Table; import javax.persistence.Temporal; import javax.persistence.TemporalType; @Entity @Table(name = "project") public class Project { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) @Column(name = "project_id") private int projectId; @Column(name = "project_name") private String projectName; @Column(name = "start_date") @Temporal(TemporalType.DATE) private Date startDate; @Column(name = "exp_end_date") @Temporal(TemporalType.DATE) private Date expectedEndDate; @OneToMany(fetch = FetchType.LAZY, mappedBy = "id.project") private Set<EmployeeProject> employeeProjects; public int getProjectId() { return projectId; } public void setProjectId(int projectId) { this.projectId = projectId; } public String getProjectName() { return projectName; } public void setProjectName(String projectName) { this.projectName = projectName; } public Date getStartDate() { return startDate; } public void setStartDate(Date startDate) { this.startDate = startDate; } public Date getExpectedEndDate() { return expectedEndDate; } public void setExpectedEndDate(Date expectedEndDate) { this.expectedEndDate = expectedEndDate; } }
Step 7: Create EmployeeProjectPK annotated class: EmployeeProjectPK is an Embeddable class.
EmployeeProjectPK.java
package com.hibernate.association.pojo; import java.io.Serializable; import javax.persistence.Embeddable; import javax.persistence.ManyToOne; @Embeddable public class EmployeeProjectPK implements Serializable { /** * */ private static final long serialVersionUID = 1L; @ManyToOne private Employee employee; @ManyToOne private Project project; public Employee getEmployee() { return employee; } public void setEmployee(Employee employee) { this.employee = employee; } public Project getProject() { return project; } public void setProject(Project project) { this.project = project; } }
Step 8: Create EmployeeProject annotated class. For employee and project association table.
EmployeeProject.java
package com.hibernate.association.pojo; import javax.persistence.AssociationOverride; import javax.persistence.AssociationOverrides; import javax.persistence.Column; import javax.persistence.EmbeddedId; import javax.persistence.Entity; import javax.persistence.JoinColumn; import javax.persistence.Table; @Entity @Table(name = "employee_proj") @AssociationOverrides({ @AssociationOverride(name = "id.employee", joinColumns = @JoinColumn(name = "employee_id")), @AssociationOverride(name = "id.project", joinColumns = @JoinColumn(name = "project_id")) }) public class EmployeeProject { @EmbeddedId private EmployeeProjectPK id = new EmployeeProjectPK(); @Column(name = "acitve") private boolean active; @Column(name = "is_deleted") private boolean deleted; public EmployeeProjectPK getId() { return id; } public void setId(EmployeeProjectPK id) { this.id = id; } public boolean isActive() { return active; } public void setActive(boolean active) { this.active = active; } public boolean isDeleted() { return deleted; } public void setDeleted(boolean deleted) { this.deleted = deleted; } public Employee getEmployee() { return getId().getEmployee(); } public void setEmployee(Employee employee) { getId().setEmployee(employee); } public Project getProject() { return getId().getProject(); } public void setProject(Project project) { getId().setProject(project); } }
Step 9. Create a HibernateUtility class: HibernateUtility class to build SessionFactory via loading Configuration file.
package com.hibernate.association.util; import org.hibernate.SessionFactory; import org.hibernate.boot.registry.StandardServiceRegistryBuilder; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; public class HibernateUtility { private static final SessionFactory sessionFactory = buildSessionFactory(); private static SessionFactory buildSessionFactory() { Configuration configuration = new Configuration(); configuration.configure(); ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder() .applySettings(configuration.getProperties()).build(); SessionFactory sessionFactory = configuration .buildSessionFactory(serviceRegistry); return sessionFactory; } public static SessionFactory getSessionFactory() { return sessionFactory; } }
Step 10. Create an EmployeeDAO class: To perform insert/update/get/delete records into the database.
EmployeeDAO.java
package com.hibernate.association.dao; import org.hibernate.Session; import org.hibernate.SessionFactory; import com.hibernate.association.util.HibernateUtility; public class EmployeeDAO { private static SessionFactory sessionFactory; static { sessionFactory = HibernateUtility.getSessionFactory(); } public static <T> Object save(T entity) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.save(entity); session.getTransaction().commit(); return entity; } public static <T> Object update(T entitye) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.merge(entitye); session.getTransaction().commit(); return entitye; } public static <T> void delete(T entity) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.delete(entity); session.getTransaction().commit(); } }
Step 11. Create an EmployeeService class: To call the methods of EmployeeDAO class to perform CRUD operations.
EmployeeService.java
package com.hibernate.association.service; import java.text.ParseException; import java.text.SimpleDateFormat; import com.hibernate.association.dao.EmployeeDAO; import com.hibernate.association.pojo.Employee; import com.hibernate.association.pojo.EmployeeProject; import com.hibernate.association.pojo.Project; public class EmployeeService { public static void main(String[] args) { Employee employee = new Employee(); SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); try { // employee details employee.setDoj(sdf.parse("2014-08-28")); employee.setFirstName("Tony"); employee.setLastName("Jha"); // project details Project project1 = new Project(); project1.setProjectName("MARS"); project1.setStartDate(sdf.parse("2013-01-01")); project1.setExpectedEndDate(sdf.parse("2015-08-28")); EmployeeDAO.save(project1); EmployeeProject employeeProject = new EmployeeProject(); employeeProject.setActive(true); employeeProject.setDeleted(false); employeeProject.setProject(project1); employeeProject.setEmployee(employee); employee.getEmployeeProjects().add(employeeProject); // calling save method of EmployeeDAO System.out.println(EmployeeDAO.save(employee)); } catch (ParseException e) { e.printStackTrace(); } } }
OUT PUT:
Hibernate: insert into project (exp_end_date, project_name, start_date) values (?, ?, ?) Hibernate: insert into employee (doj, first_name, last_name) values (?, ?, ?) Hibernate: select employeepr_.employee_id, employeepr_.project_id, employeepr_.acitve as acitve1_1_, employeepr_.is_deleted as is_delet2_1_ from employee_proj employeepr_ where employeepr_.employee_id=? and employeepr_.project_id=? Hibernate: insert into employee_proj (acitve, is_deleted, employee_id, project_id) values (?, ?, ?, ?) Employee [employeeId=101, firstName=Tony, lastName=Jha, doj=Thu Aug 28 00:00:00 IST 2014]
Download the complete example from here Source Code
Sponsored Links
Awesome tutorial. THanks. I was also expecting to see how we can use Criteria and fetch items from DB?
ReplyDeleteThank you Anand,
DeleteI have already posted a simple tutorial on using Criteria Query here >>
http://www.javamakeuse.com/2015/07/tutorial-hibernate-criteria-query.html
Keep visiting :)
please I need an exemple like this one for mapping using xml configuration.1
ReplyDeleteThank you very much! good sample project!
ReplyDelete